How to Setup Laravel Scout using Database Driver
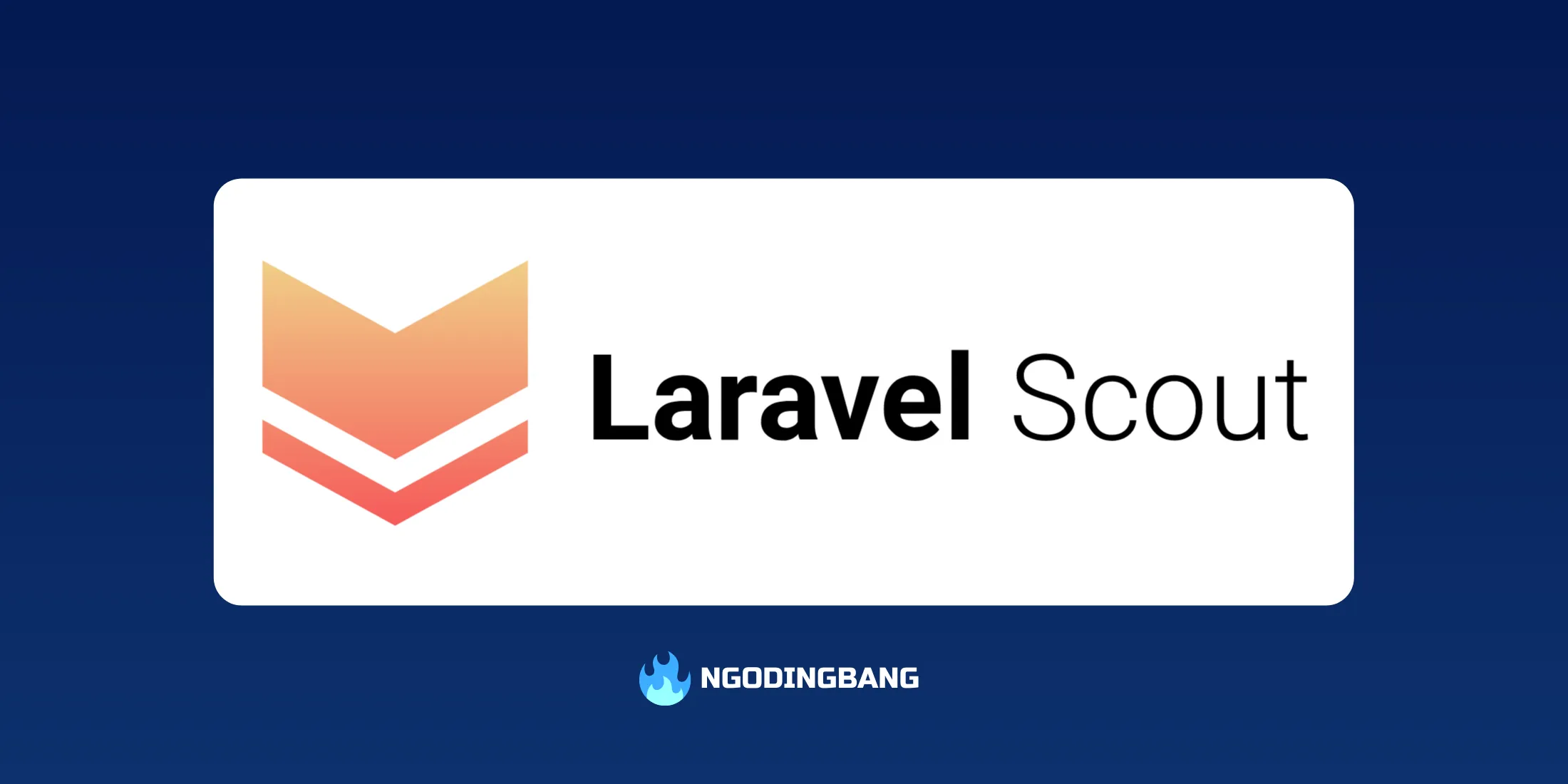
Check this article on Medium: @ngodingbang/how-to-setup-laravel-scout-using-database-driver-ad9c0ff97f4d
You may want to read this article in Bahasa Indonesia version: Cara Menggunakan Laravel Scout dengan Database Driver
TL;DR
Have you ever create an Eloquent query like this?
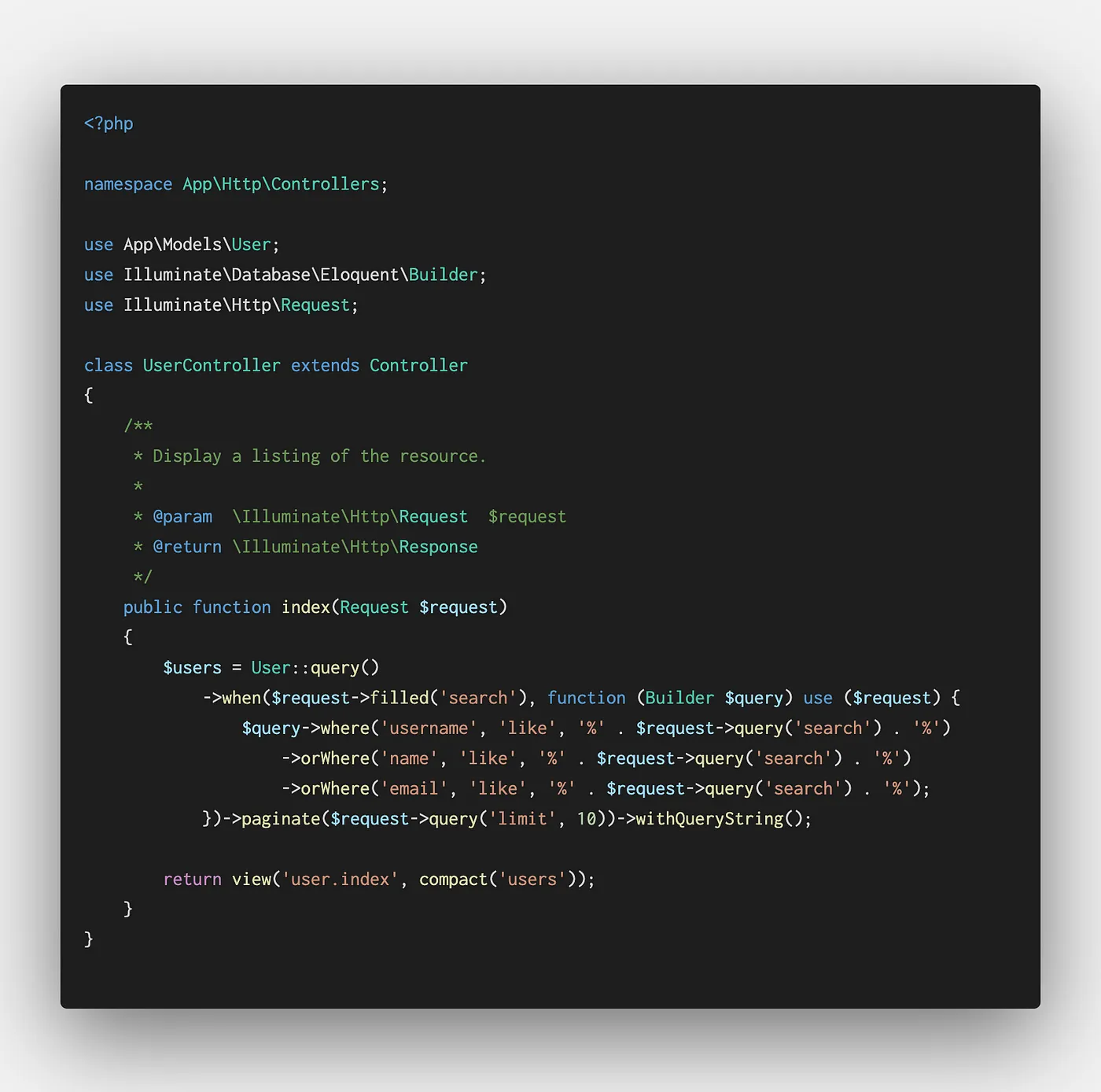
It usually happen when you try to find list of users data based on its name and email, so you’re gonna type that query into database manually using clauses “IS LIKE” like the code above. Do you know that in Laravel 9.x we can do that in much better ways using a package called Laravel Scout? If you don’t know, you better check this package out.
So basically Laravel Scout is a simple, driver-based solution for adding full-text search to your Eloquent models. This package has been introduced from Laravel 5.3, but since Laravel 9.x they introduce a new driver called “database” in their package. This driver will use where like
clauses and full-text indexes when filtering results from your existing database to determine the applicable search results for your query, something that we previously always type it in our own way. And because of its way to search the data using queries, this driver currently only supports MySQL and PostgreSQL. So for those of you who curious to know how to set it up on your application, let’s jump into this tutorial in a very few steps.
Install the Package
So before we get started, install the package first by running this command below.
composer require laravel/scout
After installation finished, publish the config/scout.php
configuration file using the vendor:publish
Artisan command php artisan vendor:publish --provider="Laravel\Scout\ScoutServiceProvider"
and change the default driver into database
. You can change it through the .env file:
SCOUT_DRIVER=database
or just directly change it at config/scout.php like these code shown below.
<?php
return [
/*
|--------------------------------------------------------------------------
| Default Search Engine
|--------------------------------------------------------------------------
|
| This option controls the default search connection that gets used while
| using Laravel Scout. This connection is used when syncing all models
| to the search service. You should adjust this based on your needs.
|
| Supported: "algolia", "meilisearch", "database", "collection", "null"
|
*/
'driver' => env('SCOUT_DRIVER', 'database'),
];
Configure the Model
Laravel Scout by default will using toArray()
method of the model to define the search index. To change this configuration, you have to override the toSearchableArray()
method on the model:
<?php
namespace App\Models;
use App\Infrastructure\Foundation\Auth\User as Authenticatable;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Notifications\Notifiable;
use Laravel\Sanctum\HasApiTokens;
use Laravel\Scout\Searchable;
class User extends Authenticatable
{
use HasApiTokens, HasFactory, Notifiable, Searchable;
/**
* Get the indexable data array for the model.
*
* @return array
*/
public function toSearchableArray()
{
return [
'username' => $this->username,
'name' => $this->name,
'email' => $this->email,
];
}
}
At this configuration, I decide to use “username”, “name”, and “email” as the attributes that can be searched by Laravel Scout. Just modify it if you have another attribute to be searched. And don’t forget to use Laravel\Scout\Searchable
trait, so Laravel Scout can run its function into your specified model.
Use it in Your Controller
And voila, you can find the User data by just doing this at your controller:
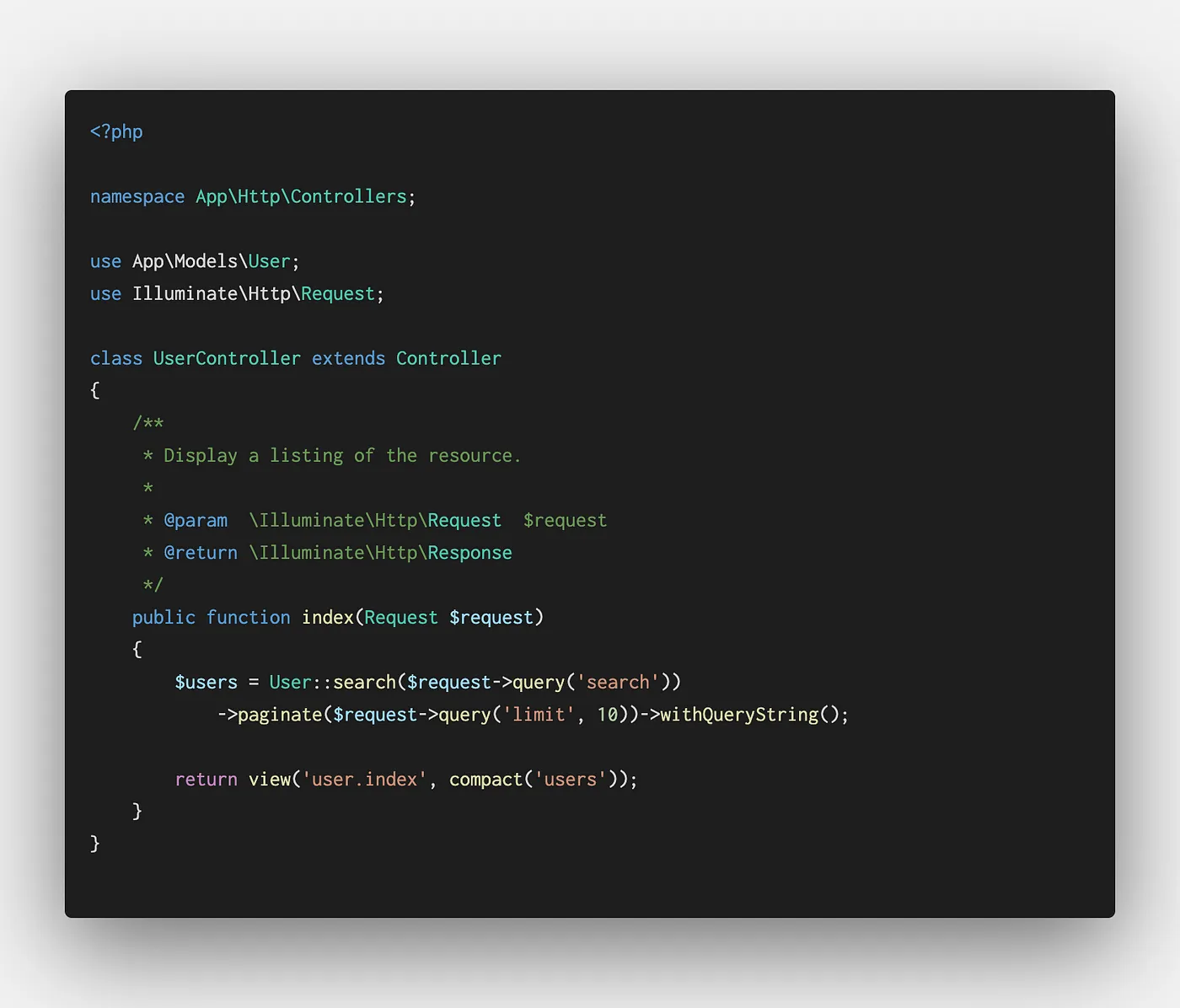
And this is what Laravel Scout will doing on your application after the process of searching data done.
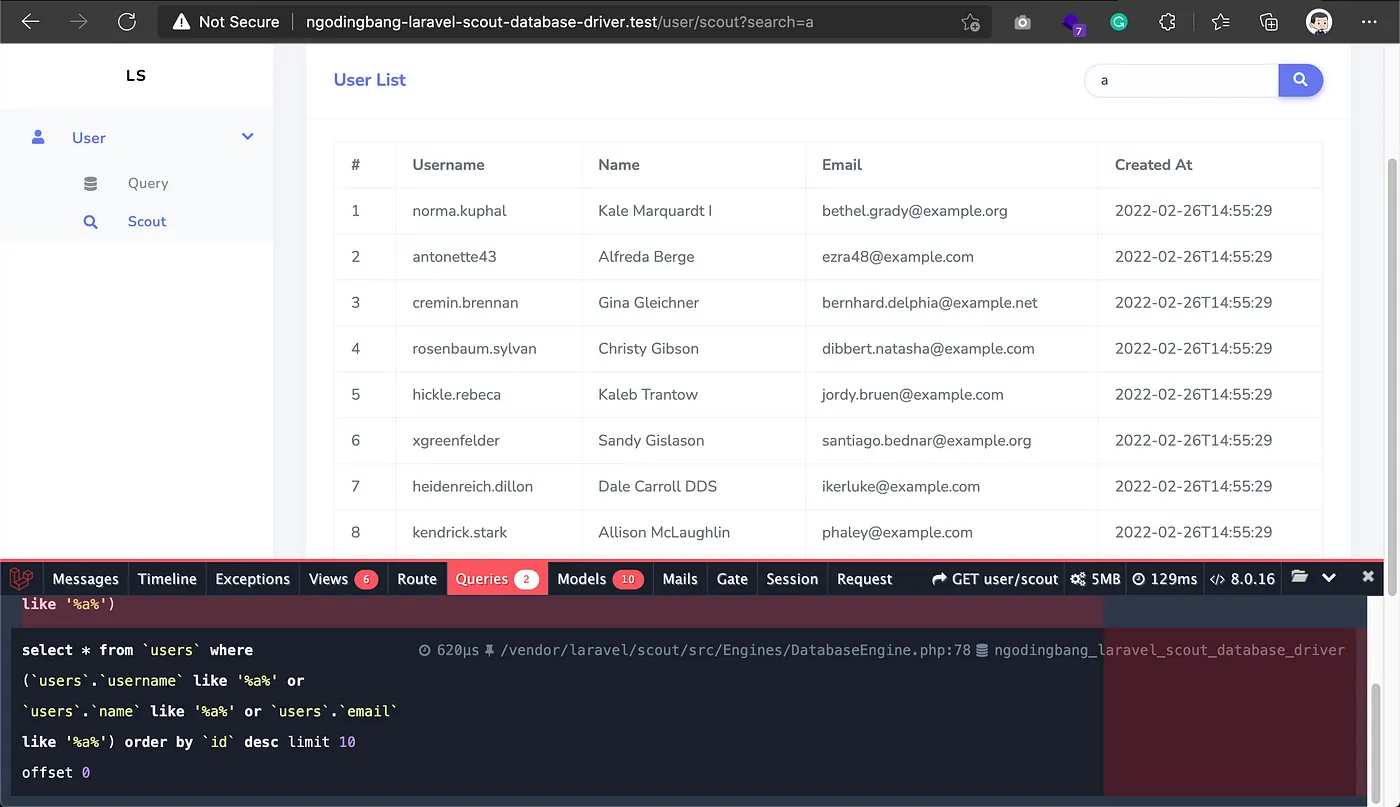
As we can see on the picture above, what Laravel Scout actually doing is just running the query that we all usually use before which is select * from
users where (
users.
username like ‘%a%’ or …
. So it just find the list of users data where they have attribute like “a” on the username, name, or email. As simple as that, but instead you don’t have to type it manually again at your controller as always.
Conclusion
The disadvantages of using database driver on Laravel Scout so far is it doesn’t work well with relationship model. It means that you can’t search the model’s data by another field that has relationship with another model, for example if you can’t search the User
data by its role name attribute from the Role
model, etc.
And at last but not least, you can check the source code example of using database driver on Laravel Scout here:
ngodingbang/laravel-scout-database-driver
Example of Laravel Application using Laravel Scout package with Database Driver.
Read more...Thanks for reading!
Source
- Laravel Scout Official Documentation (https://laravel.com/docs/9.x/scout)
- (Laravel 9: Scout with NEW Database Driver) https://www.youtube.com/watch?v=7KbJIBM5VHg