Implementing Code Coverage in GitHub Actions Using Codecov

You may want to read this article in Bahasa Indonesia version: Implementasi Code Coverage di GitHub Actions Menggunakan Codecov
Introduction
In the previous section, we discussed how to set up Continuous Integration (CI) and Continuous Deployment (CD) using GitHub Actions.
CI/CD Implementation using GitHub Actions with TypeScript and Jest
This time, we’ll continue with an equally important topic: code coverage.
Code coverage is a metric used to measure how thoroughly our application’s code is tested. By ensuring our code is covered by unit tests, we can be more confident that the application runs as expected and is free of bugs.
GitHub Actions allows us to automate the CI/CD process in a project, and one of the features in unit testing that we can leverage is integration with codecov.io. With codecov.io, we can track and visualize code coverage to ensure our application is continuously well-tested.
Implementation Steps
Setting Up GitHub Actions
The first step is to ensure that our project is already integrated with GitHub Actions as discussed in the previous article. In this session, we are using TypeScript and Jest for unit testing. Next, we will configure the workflow to run tests and send coverage data to Codecov.
Configuring GitHub Actions Workflow
Here is an example of a .github/workflows/main.yml
configuration file for a GitHub Actions workflow that automates the build and test process:
name: CI
on:
push:
branches:
- main
pull_request:
env:
NODE_VERSION: 22.11.0
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Setup Node.js
uses: actions/setup-node@v4
with:
node-version: ${{ env.NODE_VERSION }}
- name: Install dependencies
run: npm install
- name: Run test with coverage
run: npm run test -- --coverage
What differentiates the configuration above from the previous article is the use of coverage
mode. In the “Run test with coverage” section, Jest will create a coverage report in the /coverage
folder, which will then be sent to Codecov in the next step.
Configuring Codecov
Once GitHub Actions successfully generates the coverage report, we need to configure Codecov to receive and process the report. Follow these steps:
-
Sign up or log in to codecov.io.
-
Connect the GitHub repository to codecov.io
Example of a Codecov account already integrated at https://app.codecov.io. -
Find the repository name you want to configure, then go to the configuration menu as shown below.
Configuration page on Codecov (https://app.codecov.io/gh/ngodingbang/rwid-github-actions/config/general) -
Add a token to the GitHub Secrets configuration file with the name
CODECOV_TOKEN
.GitHub Actions secrets page for adding CODECOV_TOKEN (https://github.com/ngodingbang/rwid-github-actions/settings/secrets/actions/new)
In the next step, we will send coverage data from GitHub Actions to Codecov.
Sending Coverage Data to Codecov
To send coverage data to Codecov, we can add the following step to the GitHub Actions workflow:
name: CI
on:
push:
branches:
- main
pull_request:
env:
NODE_VERSION: 22.11.0
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Setup Node.js
uses: actions/setup-node@v4
with:
node-version: ${{ env.NODE_VERSION }}
- name: Install dependencies
run: npm install
- name: Run test with coverage
run: npm run test -- --coverage
- name: Upload results to Codecov
uses: codecov/codecov-action@v4
with:
token: ${{ secrets.CODECOV_TOKEN }}
fail_ci_if_error: true
This step will send the coverage data generated by Jest to Codecov.io after the tests are completed. After making changes to .github/workflows/main.yml
, don’t forget to commit the code and release a new version. For example, using the following Git commands:
git commit -m "ci: add codecov integration on github actions"
git push origin main
Verifying Coverage Results
After the GitHub Actions workflow runs, we can check the coverage report on codecov.io. Codecov will display statistics on how much code is covered by unit tests, as well as provide easy-to-understand visual reports.
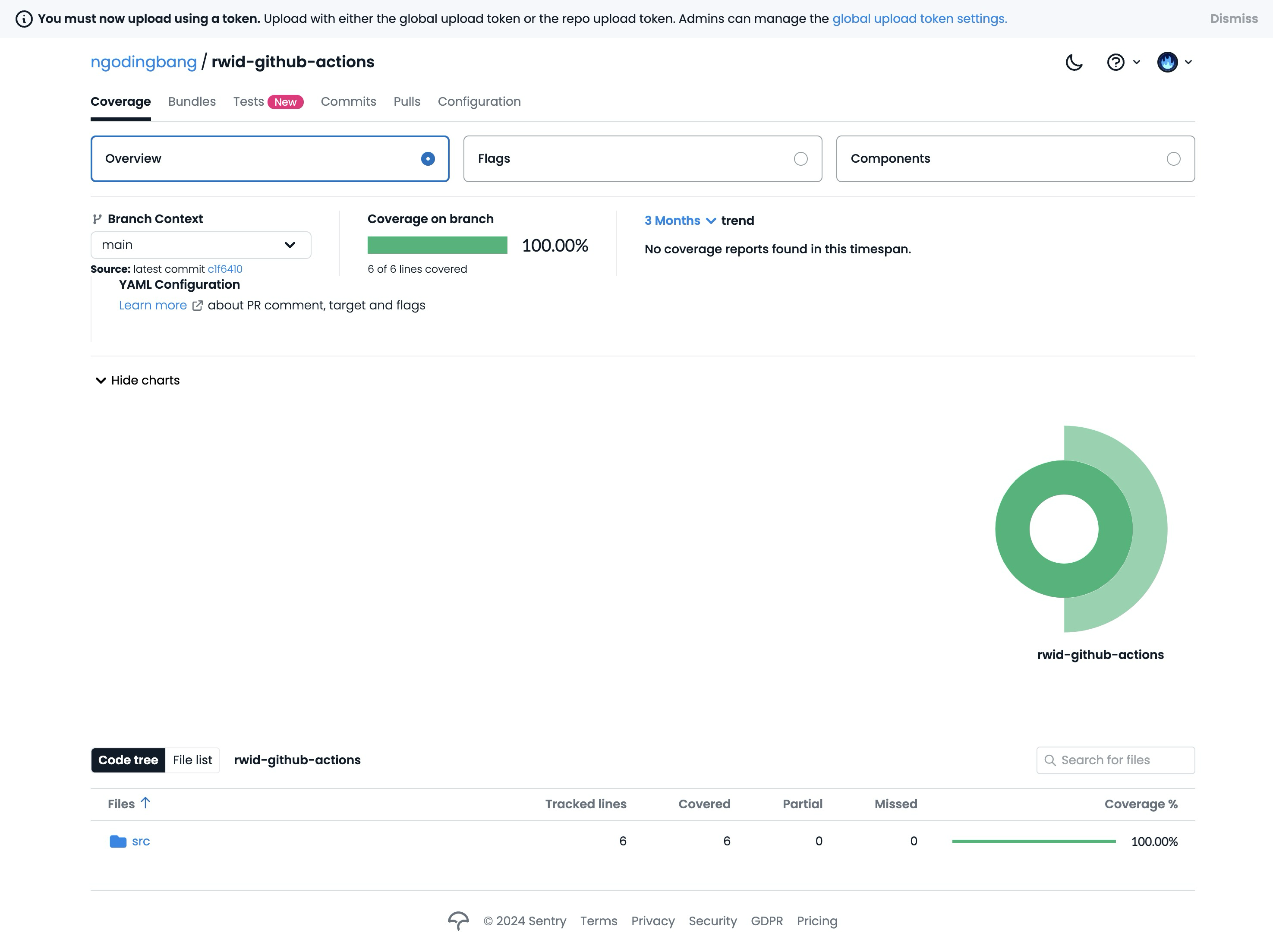
Conclusion
By using GitHub Actions and Codecov, we can automate the entire testing process and monitor code quality. Code coverage is a very useful metric to keep code well-tested and bug-free. The source code for this article can be found below.
https://github.com/ngodingbang/rwid-github-actions
Don’t forget to always update your CI/CD workflow to be more efficient and ensure the application’s quality is maintained 🔥.